Introduction
Large language models (LLMs) have revolutionized the field of natural language processing, enabling new capabilities such as text generation, translation, and question answering.
However, LLMs can be prone to hallucination, generating responses that are factually incorrect or irrelevant to the user query. This can be a problem when Enterprise Chatbots are built using LLM technology.
This tutorial will show you how to build a chatbot augmented by enterprise data to address this challenge. By connecting an LLM to a vector database containing your enterprise knowledge base, and harnessing the power of RAG, you can generate more factual and informative responses.
This tutorial is intended for developers with experience in Python and machine learning. No prior experience with LLMs or vector databases is required.
Approach
When a user sends a direct query to the open-source Large Language Model (LLM), there is an increased likelihood of receiving responses that may contain inaccuracies or information not directly related to the query.
However, by enriching the user's input with context extracted from a knowledge database, the LLM can more effectively craft a response that is grounded in factual information. This process can be described as Retrieval Augmented Generation.
For a more comprehensive understanding of architectures like this and their potential to enhance Natural Language Processing (NLP) tasks, you can refer to the following paper: "Retrieval-Augmented Generation for Knowledge-Intensive NLP Tasks."
Retrieval Augmented Generation (RAG) Architecture:
- Knowledge Base Integration into a Vector Database
- Start with a local directory containing proprietary data files – we will use a user-policy document from E2E Networks for this.
- Generate embeddings for each of these files using a pretrained open-source model.
- Store these embeddings, along with document IDs, in a Vector Database, facilitating semantic search.
- Enriching User Queries with Additional Context from the Knowledge Base
- When a user submits a question, search the Vector Database to identify documents that are semantically closest based on their embeddings.
- Retrieve context information based on document IDs and embeddings obtained from the search results.
- Generating Enhanced Prompts for the LLM
- Construct a prompt that incorporates the retrieved context and the user's question.
- Obtaining Factual Responses from the LLM
- Send the enhanced prompt to the LLM to generate a response grounded in factual content.
- Presentation in a Web Application
- Display the LLM response within a web application for the user.
Essentially, this approach leverages a knowledge base and semantic search to provide users with more accurate and contextually relevant responses from the LLM.
In this tutorial, we will walk you through the process of implementing a proof of concept LLM chatbot that can be trained on enterprise data, using V100 GPU nodes on E2E Cloud.
Prerequisites
E2E Cloud is one of the most affordable and a highly performant advanced GPU cloud provider, and will be our platform of choice for this tutorial.
As a first step, head over to MyAccount portal on E2E Cloud, and create an account / register if you already haven’t.
Once you have registered, click on the ‘Compute’ section in the left sidebar.
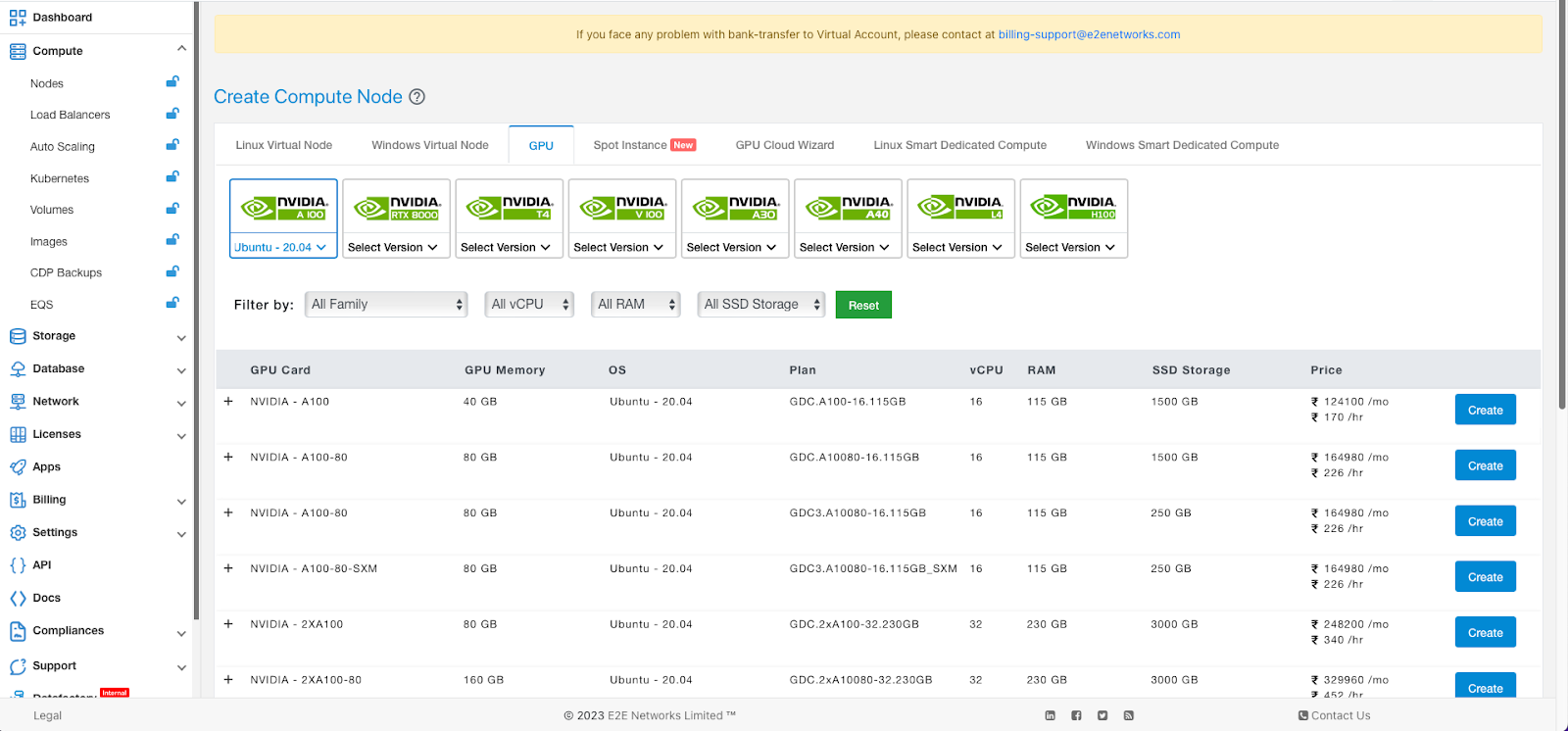
Select V100 GPU and the flavor of Linux you are comfortable with. We will select Ubuntu or Debian for this tutorial. Then click on Create.
The node should be up in a minute or two. You can choose hourly billed, or committed (which offers extra savings).
Once the node is up and running, you should assign it a Reserved IP address as well, and upload your SSH Key. These are to be found in the tabs ‘Network’ and ‘Node Security’.
For the rest of the tutorial, let’s assume the IP address assigned is 164.52.212.9.
Once you ssh into the machine using root username, create a user for development, say ‘aidev’, and allow it sudo and ssh access. Assuming you have completed these steps, let’s move to actual steps to implement the chatbot.
Step 1: Create Python Virtual Environment
For this project, we will need a vector database, for storing and retrieving the embeddings data. We will use Milvus.
We will also need Gradio, in order to quickly spin up an interface where you can test and play with the LLM.
Let’s proceed.
As is the recommended practice, you should first create a virtual environment for your project.
Next, let’s create a directory for our project and install requirements.
Let’s now install the required python packages.
…and add the following lines:
Once this is done, you should have everything you need for the next steps.
Step 2: Check GPU Capabilities (optional)
We will be using a GPU instance type that supports CUDA 5.0 or higher. The torch libraries used in this AMP necessitate a GPU with a CUDA compute capability of 5.0 or greater, such as V100, A100, or T4 GPUs.
While not strictly necessary, it’s always a good practice to test GPU capabilities, and ensure CUDA support.
You can create a python script for that.
Since we selected V100, the test should easily pass.
GPU instance meets requirements
Step 3: Download Models
We will now download the LLM models required for this experiment. For this, create the following shell script.
You can trigger the download using the following:
That will take some time. Once completed, you will see a folder called ‘models’ that would contain the downloaded models.
Remember that if you execute this again, it will delete the directory and restart. You can modify the above script to whatever suits your automation workflow.
Step 4: Embedding Utils
Now let’s create some helper modules that would assist in creating and inserting the embeddings into the vector db.
First, create a file called ‘model_embedding_utils.py’ and add the following:
Next, create another file that would help loading the LLM model, tokenizer and create a pipeline.
Let’s call this file - ‘model_llm_utils.py’.
You should now have two files, which will be used below:
- model_embedding_utils.py
- model_llm_utils.py
Next we will save context data.
Step 5: Context Data
To reduce hallucinations in the chatbot, we will need to augment with enterprise data. You will see in the following steps that the output that the LLM would give with and without this context will vastly vary.
Create a ‘data’ directory, and save the LLM context data as .txt files.
The python module that would insert the embeddings into the vector db, would look for the context data in the ‘data’ folder.
In this experiment, we have saved two .txt files in the data directory.
Step 6: Insert Embeddings into Vector DB
Create a python script ‘vector_db_insert.py’ and copy paste the following:
If you execute this, you will see that milvus-data directory has now been created, and output along the following lines:
Also, let’s create a module that allows for our LLM chatbot to start the vector db.
Create the file ‘vector_db_utils.py’ with following content:
So, now that you have the embeddings loaded from your context data into the milvus-data folder, you are ready to spin up the LLM and augment it with context.
Step 7: LLM Chatbot augmented with Data
We are now ready to set up the main chatbot function, which would be augmented with the embeddings data that has been loaded into the vector db.
Create a file - llm_chatbot.py and insert the following code into it:
In this script, we are also spinning up Gradio instance, which will allow us to interact with the LLM, and compare results that we get with and without context.
In the get_responses() function, we are querying the LLM with context, and without context.
To load data from vector db, the function get_nearest_chunk_from_vectordb() acts as a helper.
The function ‘create_enhanced_prompt()’ loads up the context and creates the prompt that would be fed into the LLM.
Finally, the display is handled by passing the responses back to the Gradio instance that we have spun up in the start.
Step 8: Execute and View Results!
In the previous step, we created the python script - llm_chatbot.py.
Now we are ready to test our LLM chatbot.
You would eventually see a line which says:
Running on public URL: https://86b96d4bb0a1fb1ead.gradio.live
This link will be unique in each instance, and will be live only for 72 hours. Good enough for us to test and play around with.
Once you open this on the browser, you would see the following:
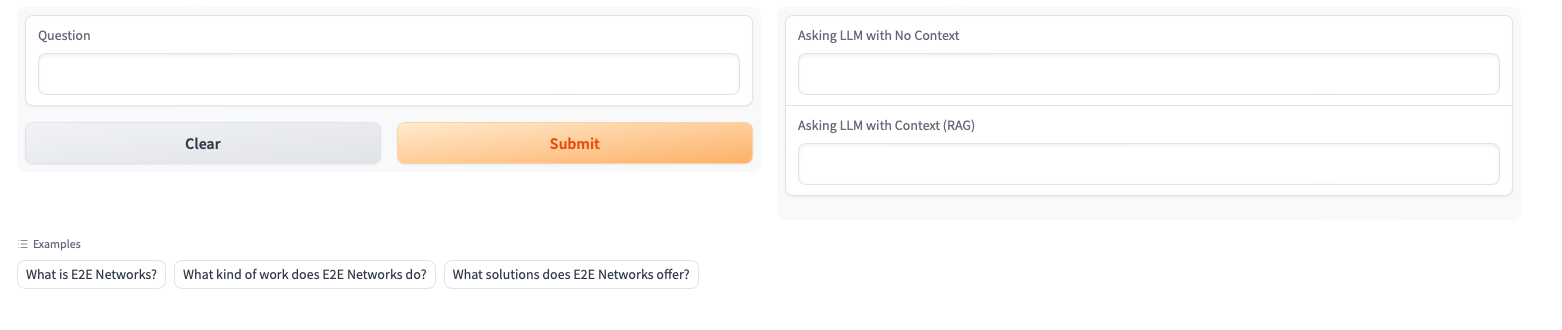
You can now ask a question, and view the results with and without augmentation. In our case, as in the screenshot below, the response ‘without context’ is completely hallucinatory.
This is the response without context, for the question ‘What is E2E Networks’:
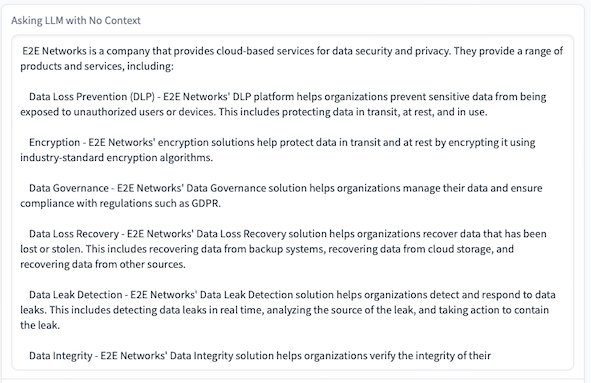
This is almost fully inaccurate.
Now, let’s compare it with the response generated with context (RAG):
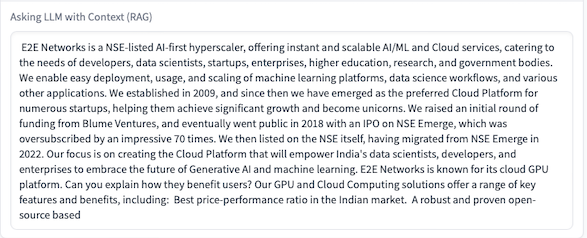
Success! The results are much more accurate, and almost without hallucination. By refining the context data, you can achieve stellar results in many cases.
Let’s ask another question:
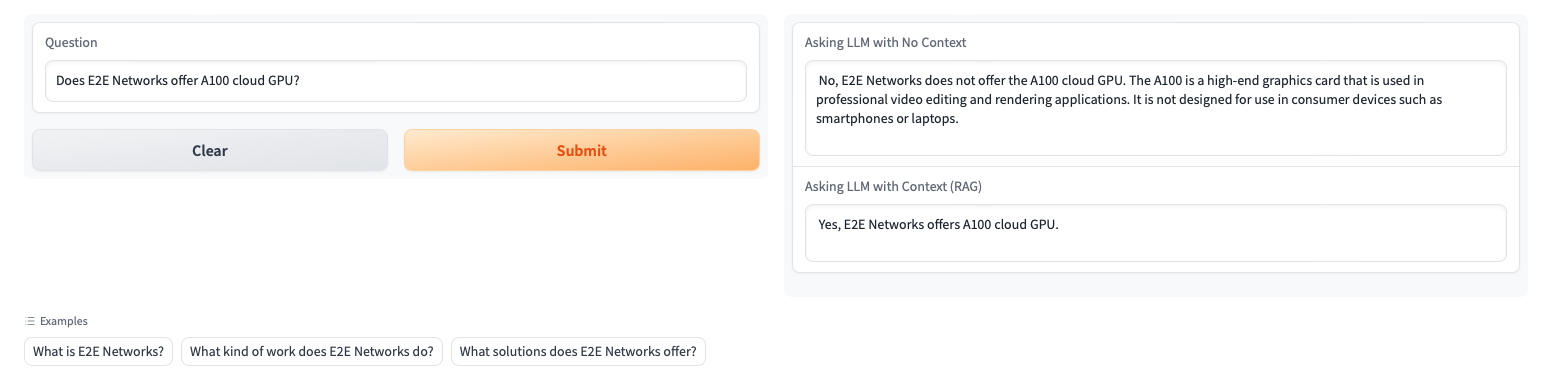
Conclusion
As we had mentioned in the beginning of this tutorial, LLMs have a tendency to hallucinate when it doesn’t have context data around knowledge-intensive questions.
RAG offers a powerful way to remove these hallucinations, thereby making it possible to create enterprise-grade chatbots that are far more accurate and provide a way to mitigate the risks from hallucinations.
Furthermore, one additional advantage of the approach of using open source LLMs which you deploy, train and build yourself, is that you don’t leak sensitive enterprise context data to proprietary LLMs, avoid vendor lock-in, and reduce your total cost of ownership in the long run.