What are neural networks?
Neural networks are a type of artificial intelligence that is modeled after the human brain. They are composed of interconnected nodes, or neurons, which are connected to each other in various ways. Each neuron is responsible for a specific task, such as pattern recognition or memory recall. Neural networks can recognize patterns and make decisions based on those patterns, and are able to learn from experience. They are used in a wide range of applications, including image recognition, natural language processing, and robotics.
Being a subset of machine learning, they are at the heart of deep learning algorithms. They are composed of node layers, containing an input layer, one or more hidden layers, and an output layer. Each node connects to another and has an associated weight and threshold value, that node is activated, sending data to the next layer of the network. Otherwise, no data is passed along to the next layer of the network.
Different types of neural networks are used in several use cases. For example: recurrent neural networks are commonly used for natural language processing and speech recognition whereas convolutional neural networks are often utilized for classification and computer vision tasks. Prior to CNNs, manual, time-consuming feature extraction methods were used to identify objects in images. However, convolutional neural networks now provide a more scalable approach to image classification and object recognition tasks, leveraging principles from linear algebra, specifically matrix multiplication, to identify patterns within an image. That said, they can be computationally demanding, requiring GPUs to train models.
CNNs- History and Evolution
The concept of convolutional neural networks (CNNs) dates back to the late 1980s, when Yann LeCun and colleagues at AT&T Bell Labs developed the first convolutional neural network for recognizing handwritten digits. This concept was later refined by researchers who developed the Neocognitron in 1980 & the widely used backpropagation algorithm for training neural networks.
In the late 1990s, CNNs began to be used more widely for tasks such as image recognition, object detection and natural language processing. The breakthrough came in 2012, when some of the prestigious researchers won the ImageNet Large-Scale Visual Recognition Challenge (ILSVRC) using a deep convolutional neural network. This success was followed by a number of similar breakthroughs in the field of computer vision, including the development of faster and more efficient CNNs, such as the ResNet architecture. In recent years, CNNs have been used for a wide range of tasks, including medical image analysis, natural language processing, autonomous vehicles, and robotics.
Why Convolutional Neural Networks?
A ConvNet is able to successfully capture the spatial and temporal dependencies in an image through the application of relevant filters. The architecture performs a better fitting to the image dataset due to the reduction in the number of parameters involved and the reusability of weights. In other words, the network can be trained to understand the sophistication of the image better. It is a class of deep neural networks that is commonly applied for analyzing visual imagery. They can also be used for other data analysis or classification problems. Most generally, we can think of a CNN as an artificial neural network that has some type of specialization for being able to pick out or detect patterns and make sense of them. This pattern detection is what makes CNN so useful for image analysis so if a CNN is just some form of an artificial neural network what differentiates it from just a standard multi-layer perceptron or MLP.
How do convolutional neural networks work?
Convolutional nets mainly work on image data and apply the so called convolutional filters so a typical confident architecture looks like the below.
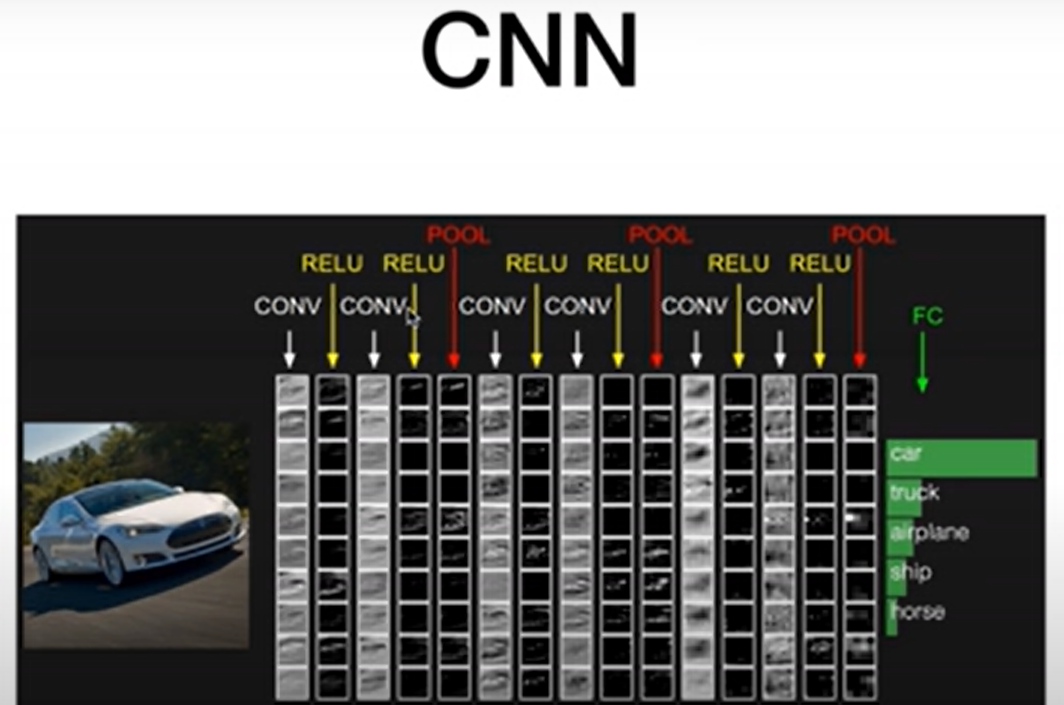
We have our image and different convolutional layers, optional activation functions followed by so-called pooling layers and these layers are used to automatically run some features from the images and then at the end we have one or more fully connected layers for the actual classification tasks.

These convolutional filters work by applying a filter kernel to our image so we put the filter at the first position as the input image and then we compute the output value by multiplying and summing up all the values and then we write the value into the output image.
In other words, a convolutional layer combines two parts and generates a function that expresses how one alters the other. Recall, if you are familiar with neural networks, that they have inputs which are fed through a layer that has weights. If you take a look at this from a convolution perspective, such a layer will have weights - and it evaluates how many inputs "alter", or "trigger" these weights.
Then, by adapting the weights during optimization, we can teach the network to be "triggered" by certain patterns present in the input data. Indeed, such layers can be taught to be triggered by certain parts that are present in some input data, such as a nose, and relate it to e.g. output class "human" (when seen from the whole network).
Since Convnets work with a kernel that is slided over the input data, they are said to be translation invariant - meaning that a nose can be detected regardless of size and position within the image. It is why ConvNets are way more powerful for computer vision problems than classic MLPs.
Why is PyTorch implemented in CNN?
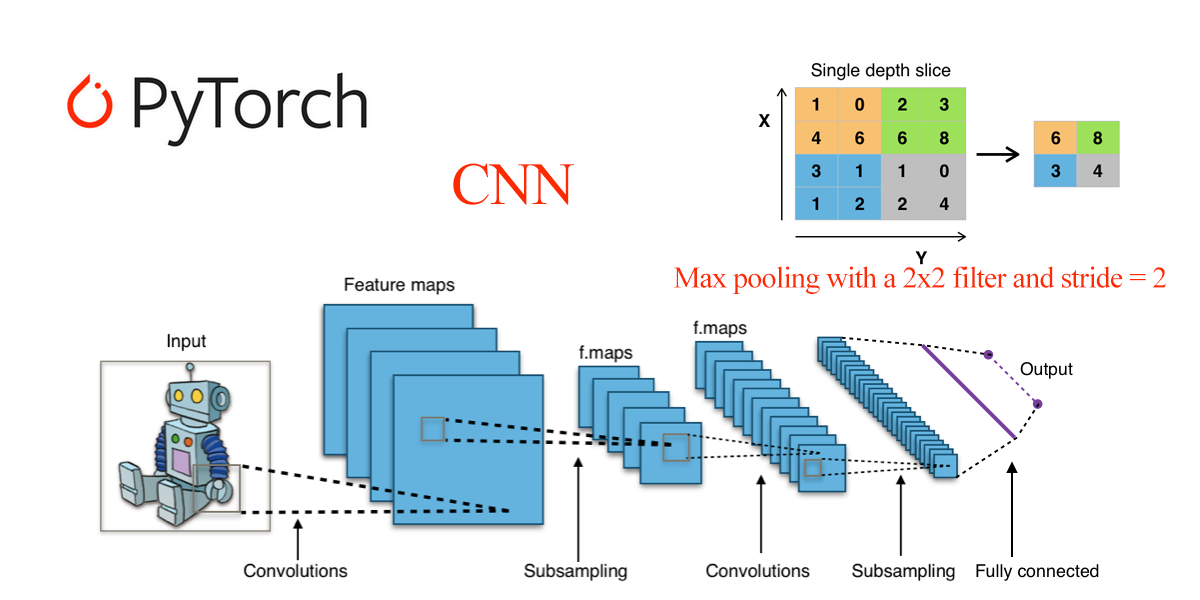
PyTorch is a powerful open-source deep learning framework that can be used to develop and deploy Convolutional Neural Networks (CNNs) in production. CNNs are a type of deep learning algorithm that can be used to analyze and classify images, videos, and other types of data. PyTorch CNNs can be used for image classification, object detection, and other computer vision tasks. They can also be used for natural language processing, speech recognition, and other tasks involving text or audio data. PyTorch CNNs are easy to use and have a high level of accuracy and performance.
When deploying PyTorch CNNs in production, it is important to consider the hardware requirements. PyTorch typically requires a GPU with CUDA support and a minimum of 8GB of RAM.
Additionally, developers must consider the software requirements, such as the version of Python and the appropriate PyTorch packages.
It is also important to consider the deployment environment, such as the cloud platform or onsite hardware setup.
Finally, developers should consider the security measures required to protect the data. When deploying PyTorch CNNs in production, it is important to perform testing and validation.
How is PyTorch implemented in neural networks?
PyTorch is used to implement Convolutional Neural Networks (CNNs) by defining the layers of the network, performing the convolution operations, and applying various activation functions. The core of the network is a convolutional layer, which takes an input image and applies a set of convolutional filters to it. These filters extract features from the input image, like edges and curves, and can be used to identify objects in the image. The output of the convolutional layer is then fed into a pooling layer, which reduces the size of the feature map by aggregating or combining the features. The result of the pooling layer is then passed through a fully-connected layer, which is a regular neural network that is used to make predictions based on the features extracted from the image.
The output of the fully-connected layer is passed through an activation function, which determines the final output of the CNN.
Steps to implement PyTorch in CNN:
1. Install PyTorch: Install the latest version of PyTorch using pip or conda depending on your system.
2. Load the Dataset: Load the dataset you wish to use for training your CNN.
3. Preprocess the Data: Preprocess the data by performing transformations such as normalization, scaling, and augmentation.
4. Define the Model: Define the CNN architecture you wish to use for your task.
5. Train the Model: Train the model using an optimizer and a loss function.
6. Evaluate the Model: Evaluate the model on the test set and measure its performance.
7. Fine-tune the Model: Fine-tune the model by adjusting its hyperparameters and tuning the architecture.
8. Deploy the Model: Deploy the model in production and serve predictions.
PyTorch CNN in Production
PyTorch offers a number of tools that can be used to evaluate, deploy, and maintain CNNs in production. These tools help to ensure that models are deployed quickly, accurately, and securely. The first step in deploying a PyTorch CNN in production is to create a model. This can be done in a variety of ways, including using pre-trained models, or training a model from scratch on a custom dataset. Once the model has been created, it can be evaluated for accuracy and performance. PyTorch provides a number of evaluation metrics, including accuracy and AUC, as well as tools for visualizing and debugging models. Once the model is evaluated, it can be deployed to production.
PyTorch provides a number of ways to deploy models, including using cloud solutions such as frameworks such as TensorFlow Serving and OpenVINO. Once the model is deployed, PyTorch also provides tools for monitoring and maintaining the model, such as performance metrics and automated alerts. Finally, the deployed model can be monitored for performance in production. PyTorch provides a number of tools for monitoring performance, including metrics such as accuracy, AUC, precision and recall. Additionally, PyTorch provides tools for debugging, such as visualizing models, weights and activations, and examining feature maps.
Deploying a PyTorch CNN in production requires a few steps:
1. Preparing your data: Depending on the size of your dataset, it may need to be split into smaller batches. Additionally, you will need to scale and normalize your data.
2. Training your model: PyTorch provides a number of built-in optimizers and loss functions. You can also use data augmentation to increase the accuracy of your model.
3. Evaluating your model: Once your model is trained, you can evaluate its performance on a test set. This will give you an indication of how well the model will perform on unseen data.
4. Deploying your model: PyTorch provides several deployment options, including direct deployment to the cloud or a local machine. You can also use a containerization solution such as Docker to deploy your model.
5. Monitoring and updating your model: You should monitor your model's performance in production, and update your model as needed. PyTorch provides tools for monitoring metrics such as accuracy and recall.
How can you deploy PyTorch/CNNs on E2E Cloud?
Using E2E Cloud Myaccount portal -
- First login into the myaccount portal of E2E Networks with your respective credentials.
- Now, Navigate to the GPU Wizard from your dashboard.
- Under the “Compute” menu extreme left click on “GPU”
- Then click on “GPU Cloud Wizard”

- For NGC Container Pytorch click on “Next” under the “Actions” column.
- Choose the card according to requirement, A100 is recommended.

Now, Choose your plan amongst the given options.
- Optionally you can add SSH key (recommended) or subscribe to CDP backup.
- Click on “Create my node”
- Wait for few minutes and and confirm that node is in running state

- Now, Open terminal on your local PC and type the following command
ssh -NL localhost:1234:localhost:8888 root@<your_node_ip>

- The command usually will not show any output which represents the command has run without any error.
- Go to a web browser on your local PC and hit the url:http://localhost:1234/
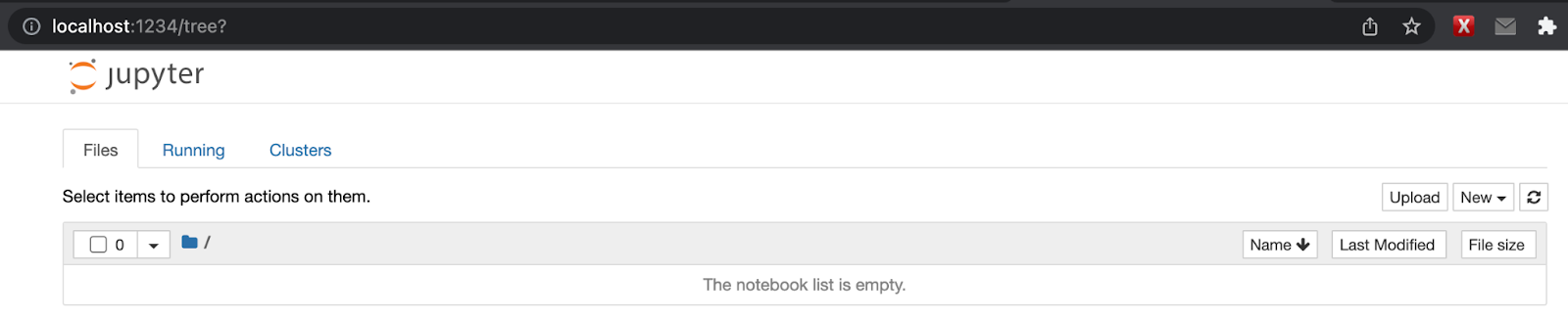
- Congratulations! Now you can run your python code inside this jupyter notebook which has Pytorch and all the libraries frequently used in machine learning preconfigured.
- To get the most out of GPU acceleration use RAPIDS and DALI which are already installed inside this container.
- RAPIDS and DALI accelerate the tasks in machine learning apart from the learning also like data loading and preprocessing.
This article covered the PyTorch implementation of a simple CNN. The reader is encouraged to play around with the network architecture and model hyperparameters to increase the model accuracy even more.
We hope we were able to guide you through the implementation of PyTorch on E2E Cloud. As you know, E2E Cloud is an accelerated cloud computing platform for training your ML models.
You can contact us for your free trial: sales@e2enetworks.com
Reference Links:
https://www.youtube.com/watch?v=IKOHHItzukk